This article is a guide on using DeepFakeAI using PHP. This guide will go through the details of creating a simple client that will generate a video using the FakeAI API so that you can generate a video on your website.
Before we get started, please make sure you know the basics of PHP, JS and how APIs work so that you know what is going on. Please also ensure that you have bought developer minutes on the FakeAI DApp so that you can actually use the API and generate videos.
Get API Keys
First, get the API keys from the FakeAI Dapp. You can do so by clicking on API Keys Tab on the Dapp and then by clicking on Generate.
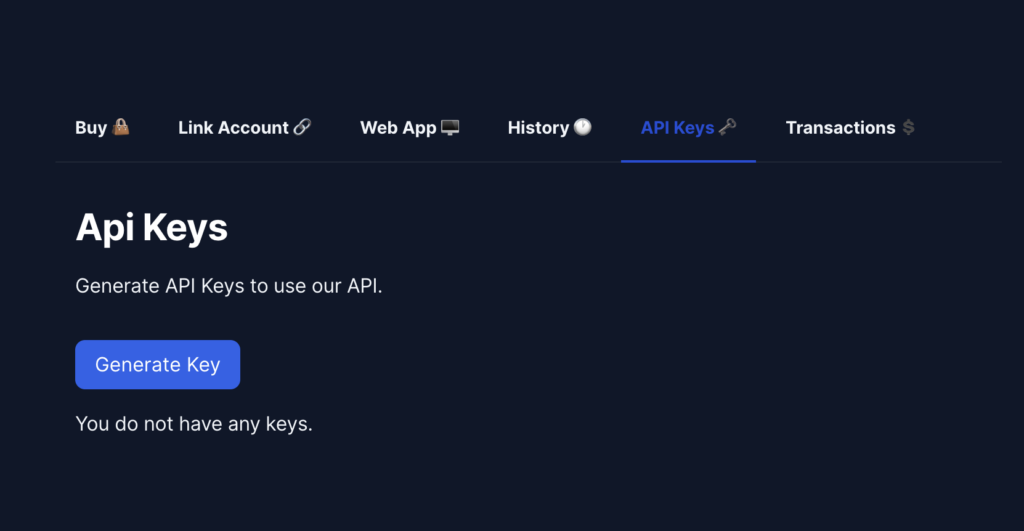
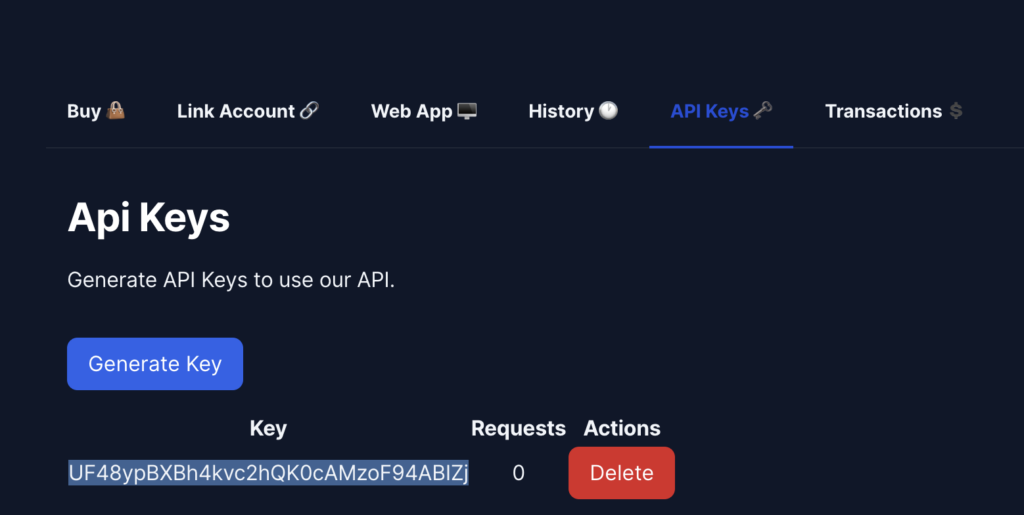
Setup Project
Create a new project in your favorite IDE, I used VSCode in my case. Then, install GuzzleHTTP using the following command.
composer require guzzlehttp/guzzle
Once it is installed, create a new file called Generate.php
.
Generation Request
In the Generate file, include the autoload.php file in the vendor folder.
require 'vendor/autoload.php';
Then, create a new variable called $key and add your key.
$key = "yourkey";
After that, create the following variables to get the parameters for generating the video.
$queryType = $_GET['type'];
$character = $_GET['character'];
$query = $_GET['query'];
Then, create a new GuzzleHTTP Client and initalize the API endpoint with it.
$client = new \GuzzleHttp\Client([
'base_uri'=>"https://os01.fakeai.io/"
]);
Now, build the query parameters for making the request using the following code and send the request using Guzzle.
$params = [
'key'=>$key,
'type'=>$queryType,
'character'=>$character,
'query'=>$query
];
$response = $client->request('GET', '/api/generate', [
'query'=>$params
]);
Then, echo out the response with the JSON header so that you can use it in Javascript.
header('Content-Type: application/json');
echo $response->getBody();
Creating User Interface & Polling Check requests
HTML Template
Create a new file index.html
and add the following html code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Generate DeepFakes</title>
</head>
<body>
</body>
</html>
After you have pasted in the template, add the following HTML code required for generating the video.
<select id="characters"></select>
<input id="query" />
<select id="type">
<option value="ask">Ask</option>
<option value="say">Say</option>
</select>
<button onclick="generate()">Generate</button>
<br />
<video id="video" controls></video>
Then, create a script tag before the end of the body tag.
Javascript Code
Then create the Javascript function to get the characters.
async function getCharacters() {
const el = document.getElementById("characters");
const request = await fetch("https://os01.fakeai.io/api/characters");
const characters = await request.json();
characters.forEach((character) => {
const option = document.createElement("option");
option.value = character.code;
option.innerText = character.name;
el.appendChild(option);
});
}
Create another function called generate()
and check(id)
to send the request to Generate.php and poll the status of the requests.
async function generate() {
const character = document.getElementById("characters").value;
const query = document.getElementById("query").value;
const type = document.getElementById("type").value;
const video = document.getElementById("video");
const request = await fetch(
`/Generate.php?character=${character}&query=${query}&type=${type}`
);
const response = await request.json();
check(response.id);
}
function check(id) {
const interval = setInterval(async () => {
const request = await fetch(`https://os01.fakeai.io/api/check/${id}`);
const response = await request.json();
if (response.status === "success") {
clearInterval(interval);
const video = document.getElementById("video");
video.src = response.video;
video.play();
}
}, 2000);
}
The two functions get the input data and send the request to Generate.php. The Generate.php file acts as a proxy so that we do not expose our keys. Then, ee get the ID from the generate function and pass it to the check function where it queries the /check endpoint on the FakeAI API every two seconds. Once the API responds with a “success” status, we remove the interval and add the video to the video tag and play it.
And last but not the least, call the following function before the end of script tag to get the characters.
getCharacters();
Final Code
The both files should be look like this at the end.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Generate DeepFakes</title>
</head>
<body>
<select id="characters"></select>
<input id="query" />
<select id="type">
<option value="ask">Ask</option>
<option value="say">Say</option>
</select>
<button onclick="generate()">Generate</button>
<br />
<video id="video" controls></video>
<script>
async function getCharacters() {
const el = document.getElementById("characters");
const request = await fetch("https://os01.fakeai.io/api/characters");
const characters = await request.json();
characters.forEach((character) => {
const option = document.createElement("option");
option.value = character.code;
option.innerText = character.name;
el.appendChild(option);
});
}
async function generate() {
const character = document.getElementById("characters").value;
const query = document.getElementById("query").value;
const type = document.getElementById("type").value;
const video = document.getElementById("video");
const request = await fetch(
`/Generate.php?character=${character}&query=${query}&type=${type}`
);
const response = await request.json();
check(response.id);
}
function check(id) {
const interval = setInterval(async () => {
const request = await fetch(`https://os01.fakeai.io/api/check/${id}`);
const response = await request.json();
if (response.status === "success") {
clearInterval(interval);
const video = document.getElementById("video");
video.src = response.video;
video.play();
}
}, 2000);
}
getCharacters();
</script>
</body>
</html>
Generate.php
<?php
require 'vendor/autoload.php';
$key = "your-key";
$queryType = $_GET['type'];
$character = $_GET['character'];
$query = $_GET['query'];
$client = new \GuzzleHttp\Client([
'base_uri'=>"https://fakeai.io/"
]);
$params = [
'key'=>$key,
'type'=>$queryType,
'character'=>$character,
'query'=>$query
];
$response = $client->request('GET', '/api/generate', [
'query'=>$params
]);
header('Content-Type: application/json');
echo $response->getBody();
End Result
If you have done everything correctly, the end result should look like this.
That’s it! You now know how to integrate DeepFakeAI in your website. If you have any issues and suggestions on improving the article please feel free to comment down below!